Back
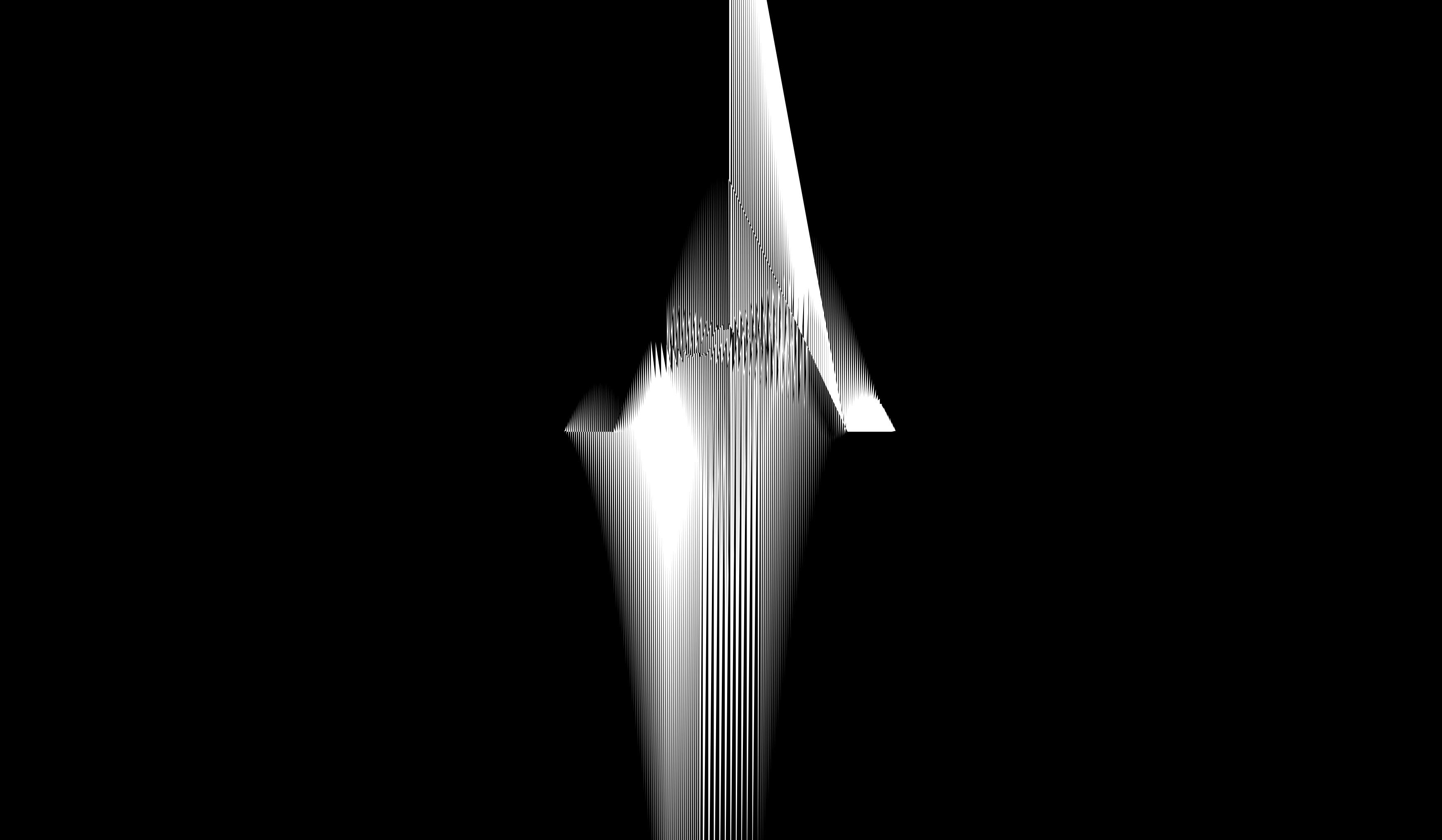
Seeking Graphic Forms Through Frequencies
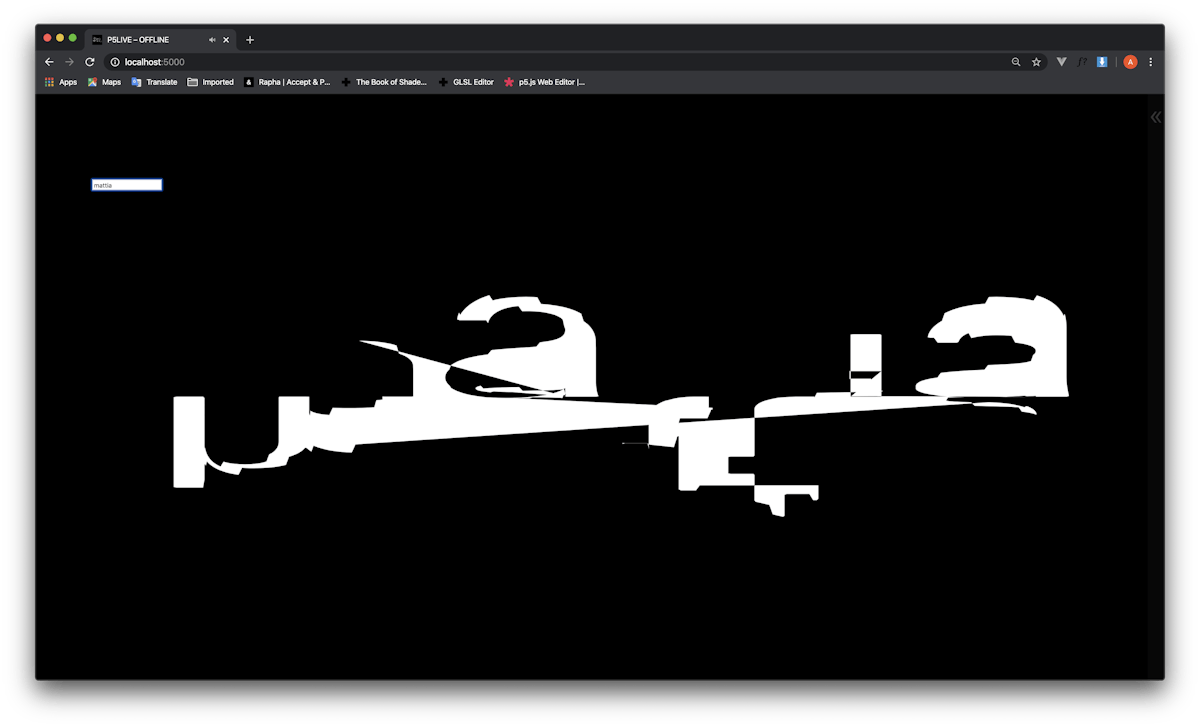
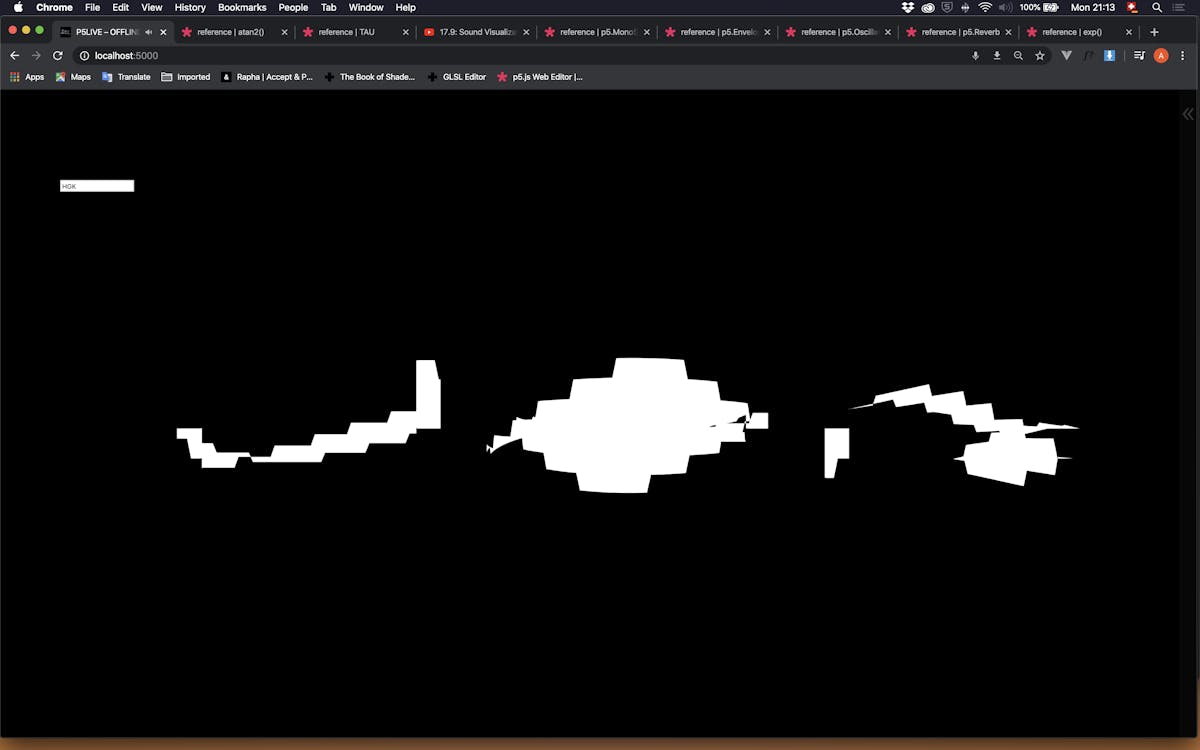
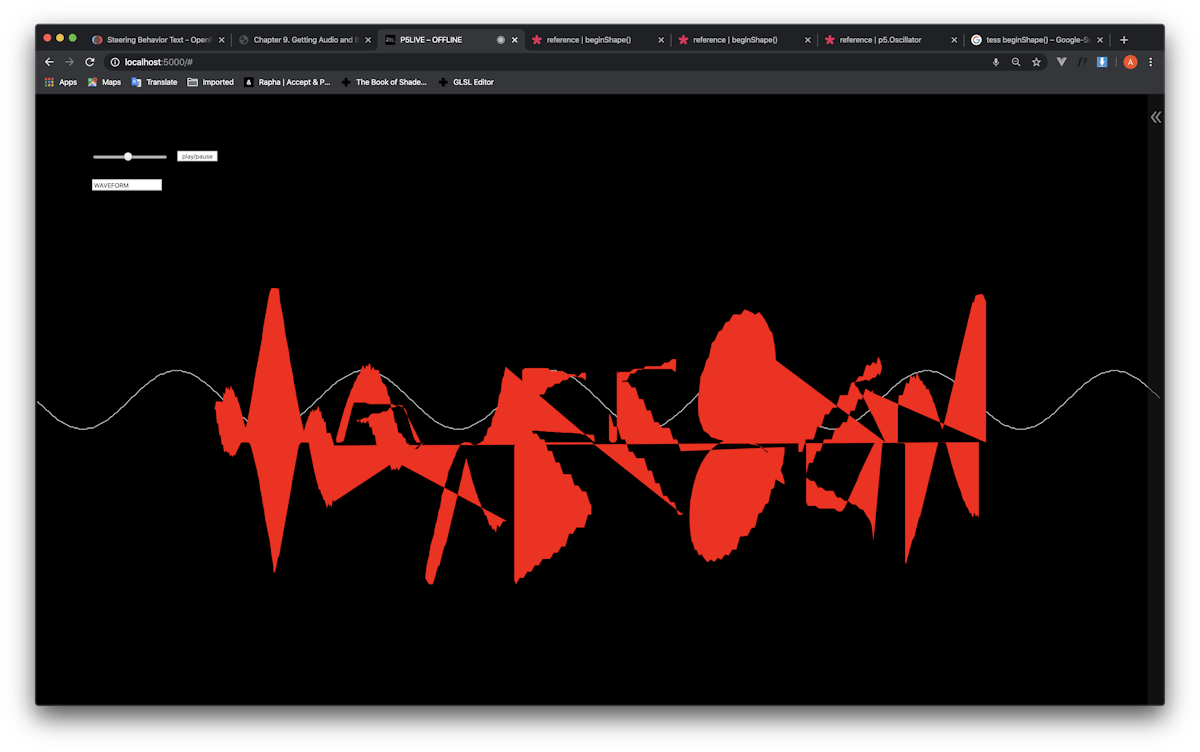
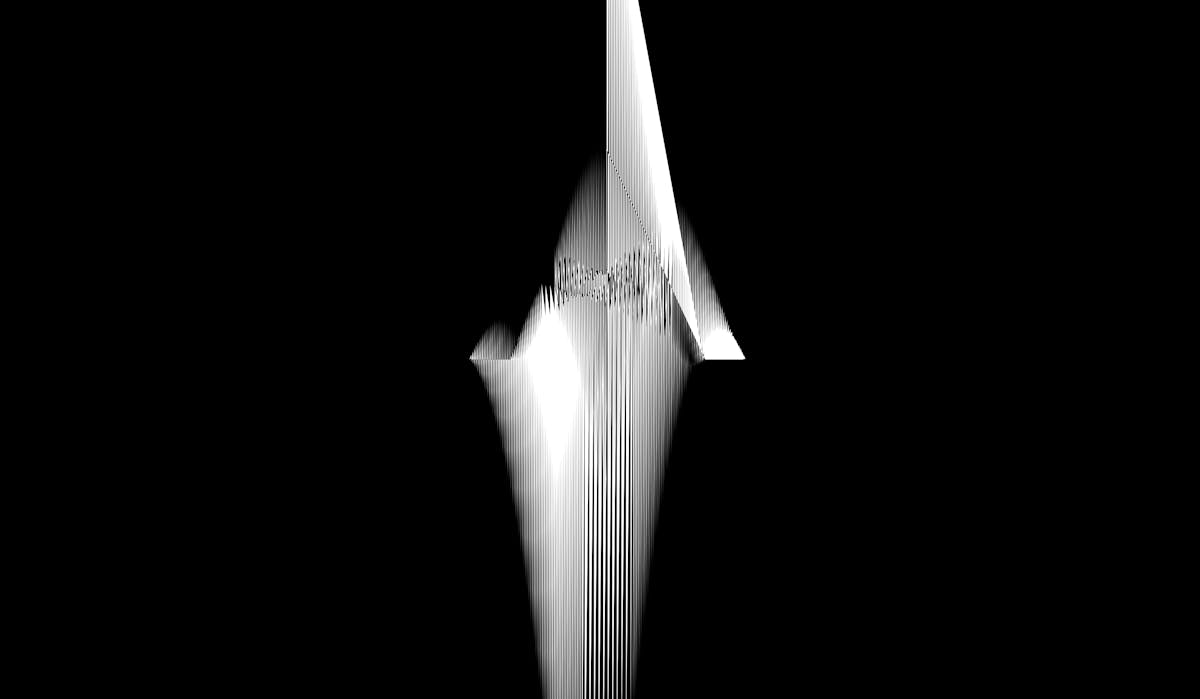
let osc, fft; var wave; let myFont; var button; var slider; var playing = false let txt, txtSize, txtOptions; let inp; function preload() { myFont = loadFont('data/fonts/Lausanne/Lausanne-300.otf') } function setup() { createCanvas(windowWidth, windowHeight); osc = new p5.TriOsc(); // set frequency and type osc.amp(0.5); fft = new p5.FFT(); osc.start(); // slider = createSlider(1, 1200, 440); // slider.position(100, 100) inp = createInput('A'); inp.position(100, 150); inp.input(updateInput); txt = 'A'; txtSize = 600; txtOptions = { //amounts of points using sampleFactor: .15, simplifyThreshold: 0 } genType() } function genType() { pts = myFont.textToPoints(txt, 0, 0, txtSize, txtOptions) bounds = myFont.textBounds(txt, 0, 0, txtSize, txtOptions); } function updateInput() { txt = inp.value(); genType() } function draw() { background(0,255); let waveform = fft.waveform(); // analyze the waveform //beginShape(); //strokeWeight(5); //for (let i = 0; i < waveform.length; i++) { // let x = map(i, 0, waveform.length, 0, width); // let y = map(waveform[i], -1, 1, height, 0); // vertex(x, y); //} //endShape(); push(); translate(width / 2, height / 2-200); translate(-bounds.w / 2, bounds.h / 2) beginShape(); // stroke(255) // noStroke() blendMode(DIFFERENCE) fill(255) strokeWeight(2); for(let i = 0; i < pts.length; i++) { let aWave = waveform[ceil(map(i, 0, pts.length, 0, waveform.length))] let aFFT = fft[ceil(map(i, 0, pts.length, 0, fft.length))] let x = map(i, 0, pts.length - 1, 0, width); vertex(pts[i].x, pts[i].y * ((aWave * TWO_PI))); vertex(pts[i].x, pts[i].y * (sin(aWave * TWO_PI))); vertex(pts[i].x, pts[i].y * (cos(aWave * TWO_PI))); } endShape(); pop(); // change oscillator frequency based on mouseX let freq = map(mouseX, 0, width, 40, 880); osc.freq(freq); let amp = map(mouseY, 0, height, 1, 0.01); osc.amp(amp); }
//TYPE REACTING ON SOUND WAVE / SINUS, TRIANGLE, SQUARE, TOOTHSAW //THESIS WORKSHOP DAY //CC ALAIN BRUSCH var wave; let myFont; var button; var slider; var playing = false let txt, txtSize, txtOptions; let inp; function preload() { myFont = loadFont('data/fonts/AkzidenzGrotesk-LightExtended.otf') // <-- YOUR LOCAL .OTF – FONTFILE } function setup() { createCanvas(windowWidth, windowHeight); background(0) wave = new p5.Oscillator(); slider = createSlider(1, 1200, 440); slider.position(100, 100) wave.setType('sine'); //change between 'sine' or 'triagle' or 'toothsaw' or 'square' wave.start(); wave.freq(440); wave.amp(0); inp = createInput('A'); inp.position(100, 150); inp.input(updateInput); txt = 'A'; txtSize = 200; txtOptions = { //amounts of points using sampleFactor: .15, simplifyThreshold: 0 } genType() button = createButton('play/pause'); button.mousePressed(toggle); button.position(250, 100) setupAudio(); } function genType() { pts = myFont.textToPoints(txt, 0, 0, txtSize, txtOptions) bounds = myFont.textBounds(txt, 0, 0, txtSize, txtOptions); } function updateInput() { txt = inp.value(); genType() } function draw() { /* audio vars: amp, ampEase, fft, waveform */ updateAudio(); wave.freq(slider.value()); clear() stroke(255); noFill(); /* waveform */ beginShape(); for(let i = 0; i < waveform.length; i++) { let freq = waveform[i] * height / 4; // (-1, 1); let x = map(i, 0, waveform.length, 0, width); curveVertex(x, height / 2 + freq); } endShape(); push(); translate(width / 2, height / 2); translate(-bounds.w / 2, bounds.h / 2) beginShape(QUAD_STRIP); noFill(); stroke(255); // fill(255) strokeWeight(1); for(let i = 0; i < pts.length; i++) { let aWave = waveform[ceil(map(i, 0, pts.length, 0, waveform.length))] let aFFT = fft[ceil(map(i, 0, pts.length, 0, fft.length))] let x = map(i, 0, pts.length - 1, 0, width); vertex(pts[i].x, pts[i].y * ((aWave * 10))); } endShape(); pop(); print(slider.value()) } function toggle() { if(!playing) { wave.amp(0.5, 1); playing = true; } else { wave.amp(0, 1); playing = false; } } /* AUDIO INIT */ let mic, fftRaw, fft = [], waveform = [], amp = 0.0, ampStereo = { l: 0.0, r: 0.0 }, ampEase = 0.0, numBins = 512, bands = 12; function setupAudio() { userStartAudio(); mic = new p5.AudioIn(); mic.start(); fftRaw = new p5.FFT(0.75, numBins); fftRaw.setInput(mic); } function updateAudio() { fftRaw.analyze(); amp = mic.getLevel() * 1000; // average mixed amplitude ampStereo.l = mic.amplitude.getLevel(0) * 500; // average left amplitude ampStereo.r = mic.amplitude.getLevel(1) * 500; // average right amplitude ampEase = ease(amp, ampEase, 0.075); // smooth 'amp' waveform = fftRaw.waveform(); // array (-1, 1) fft = fftRaw.logAverages(fftRaw.getOctaveBands(bands)); // array (0, 255) }